jQueryを使って背景画像入れ替えとカルーセル(プラグインなし)
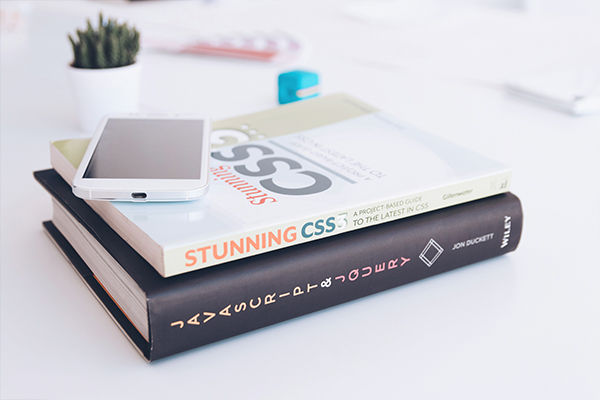
ちょっとしたギャラリー的なのに。imgで見せると幅や高さが変わってしまったり、調節してもなんか微妙ーな感じになったりするので、背景として見せています。
しかも縦横比固定なのでレスポンシブにも。
backgroundはcontainにしてますが、coverもいいよね。挙動とかデザインとか縦横比とか(縦横比って書いてあるところ)任意にどうぞっす。
jQuery
$(function() { detailimg(); $('.detaillist a:first-of-type').addClass('active'); var listcount = $('.detaillist a').length; var thumbfour = Math.ceil(listcount / 4); var nowpage = 1; if(thumbfour <= nowpage) { $('.next').css('opacity', '.1'); } $('.detaillist a').click(function(){ var swap = $(this).css('background-image').match(/https?:\/\/[-_.!~*'()a-zA-Z0-9;\/?:@&=+$,%#]+[a-z]/g); $('.detail div').fadeTo("fast", 0.5, function(){ $('.detail div').css('background-image', 'url(' + swap +')').fadeTo("fast", 1); }); var caption = $(this).children('span').text(); $('.detail + .caption').text(caption); $('.detaillist a').removeClass('active'); $(this).addClass('active'); }); $('.next').click(function(){ if(thumbfour <= nowpage) { return false; } else { var nextpage = nowpage + 1; $('.detaillist a.page' + nowpage).fadeOut(function(){ $('.detaillist a.page' + nextpage).fadeIn(); }); $('.prev').css('opacity', '1'); nowpage = nowpage + 1; if(thumbfour <= nowpage) { $('.next').css('opacity', '.1'); } } }); $('.prev').click(function(){ if(nowpage <= 1) { return false; } else { var prevpage = nowpage - 1; $('.detaillist a.page' + nowpage).fadeOut(function(){ $('.detaillist a.page' + prevpage).fadeIn(); }); $('.next').css('opacity', '1'); nowpage = nowpage - 1; if(nowpage <= 1) { $('.prev').css('opacity', '.1'); } } }); }); $(window).resize(function(){ detailimg(); }); function detailimg() { var imgwidth = $('.detail').width(); var imgheight = $('.detail').width() * 0.58; //縦横比 $('.detail div').css({height: imgheight + 'px'}); var listwidth = $('.detaillist a').width(); var listheight = $('.detaillist a').width() * 0.58; //縦横比 $('.detaillist').css({height: listheight + 'px'}); $('.detaillist a').css({height: listheight + 'px'}); }
CSS
.detail { width: 100%; margin: 2rem 0; } .detail > div { width: 100%; background-size: contain; background-position: center; background-repeat: no-repeat; background-color: #eee; } .caption { height: 2.7rem; font-size: 1.4rem; } .detaillist { position: relative; display: -webkit-box; display: -ms-flexbox; display: -webkit-flex; display: flex; -webkit-box-pack:justify; -ms-flex-pack:justify; -flex-pack:justify; -ms-flex-wrap:wrap; flex-wrap: wrap; -webkit-justify-content: flex-start; justify-content: flex-start; margin: 1rem 0 4.5rem; padding: 0 2rem; overflow-y: hidden; } .detaillist a { display: block; width: 23%; height: auto; margin-right: 2.66%; border: 1px solid #eee; background-size: cover; background-position: center; background-repeat: no-repeat; background-color: #fff; transition: all 0.15s linear; cursor: pointer; } .detaillist a span { position: absolute; bottom: -4rem; color: transparent; } .detaillist a:nth-of-type(4n) { margin-right: 0; } .detaillist a:hover { opacity: 0.7; } .detaillist a:not(.page1) { display: none; } .detaillist .active { border: 1px solid #000; } .prev, .next { display: block; position: absolute; z-index: 5; width: 2rem; height: 100%; left: 0; color: #fff; font-size: 1.7rem; cursor: pointer; background-color: #666; } .prev span, .next span { position: absolute; top: 50%; left: 50%; -webkit-transform: translate(-50%, -50%); -moz-transform: translate(-50%, -50%); -ms-transform: translate(-50%, -50%); transform: translate(-50%, -50%); } .prev { opacity: 0.1; } .next { left: auto; right: 0; } .prev:hover, .next:hover { color: #fff; background-color: #666; }
HTML
<div class="detail"> <div style="background-image: url(http://memo.exp.jp/wp-content/uploads/2018/04/css-js-1.jpg)"></div> </div> <p class="caption">キャプション1</p> <div class="detaillist"> <span class="prev"><span><</span></span> <span class="next"><span>></span></span> <a style="background-image: url(http://memo.exp.jp/wp-content/uploads/2018/04/css-js-1.jpg)" class="page1"><span>キャプション1</span></a> <a style="background-image: url(http://memo.exp.jp/wp-content/uploads/2018/02/css-1.jpg)" class="page1"><span>キャプション2</span></a> <a style="background-image: url(http://memo.exp.jp/wp-content/uploads/2017/11/mic_mixer-1.jpg)" class="page1"><span>キャプション3</span></a> <a style="background-image: url(http://memo.exp.jp/wp-content/uploads/2017/11/tablet_smartphone-1.jpg)" class="page1"><span>キャプション4</span></a> <a style="background-image: url(http://memo.exp.jp/wp-content/uploads/2017/02/a16964955aee_s-1.jpg)" class="page2"><span>キャプション5</span></a> </div>